HTD
Android Library FiveStarsView has been released. 본문
I released FiveStarsView which is a custom view for a touchable star rating view with customizable stars. With FiveStarsView, you can draw a custom view with touchable five stars, apply Two-way data binding to the view, and modify the image, color, size, and the like of those stars.
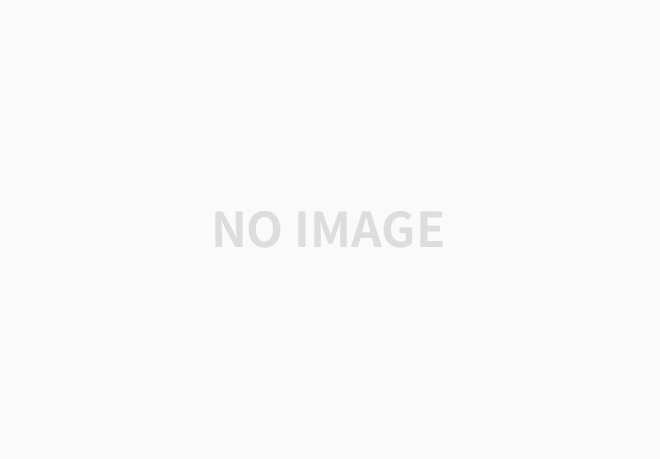
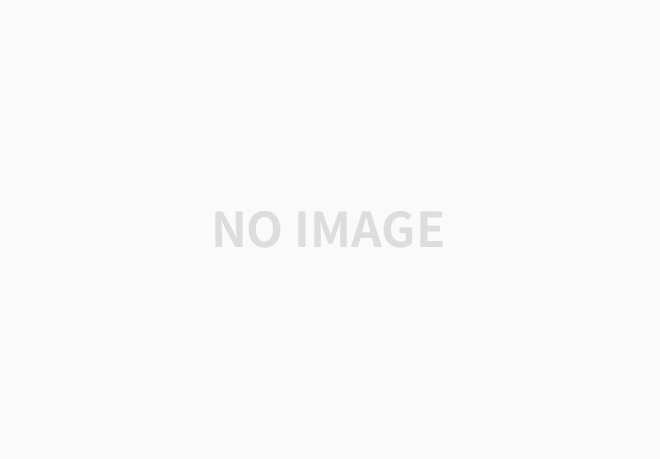
And I'd like to talk about the contents below:
- How the custom view's implemented
- Two-way data binding with custom view
How the custom view's implemented
At first, overriding view class(e.g. FrameLayout) is needed to make a custom view class. If you want to write a custom text view class, you can write a class implementing TextView. In same way I wrote a class named FiveStarsView implementing FrameLayout.
After that I wrote a layout xml of the custom view and make the layout inflated when the custom view class's initialized.
// Note that I used @JvmOverloads annotation to prevent the class from missing constructor error which occured when the class's called from java code.
class FiveStarsView @JvmOverloads constructor(
context: Context,
attrs: AttributeSet? = null,
defStyle: Int = 0
) : FrameLayout(context, attrs, defStyle) {
private val view = inflate(context, R.layout.layout_five_stars_view, this)
}
Implementating Attributes of View
To implement attributes of the view, I added a <declare-styleable>
resource named attrs_fivestarsview.xml
in /res/values
as below.
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="FiveStarsView">
<attr name="fiveStarsView_starRating" format="float"/>
<attr name="fiveStarsView_starSize" format="dimension"/>
<attr name="fiveStarsView_starColor" format="color"/>
<attr name="fiveStarsView_starMargin" format="dimension"/>
<attr name="fiveStarsView_changeable" format="boolean"/>
<attr name="fiveStarsView_filledStarDrawable" format="reference"/>
<attr name="fiveStarsView_outlineStarDrawable" format="reference"/>
</declare-styleable>
</resources>
There are many formats for :
- integer
- string
- boolean
- float
- color
- dimension
- reference: represents a reference to a resource(e.g. image, layout)
- enum
<attr name="attributeName" format="enum">
<enum name="value1" value="0" />
<enum name="value2" value="1" />
...
</attr>
- attr
<attr name="attributeName" format="flags">
<flag name="flag1" value="0" />
<flag name="flag2" value="1" />
...
</attr>
Then you can use the view in xml as the code below.
<com.hanbikan.fivestarsview.FiveStarsView
android:id="@+id/five_stars_view"
android:layout_width="250dp"
android:layout_height="70dp"
app:fiveStarsView_starSize="100dp"
app:fiveStarsView_starMargin="10dp"
app:fiveStarsView_starColor="@color/yellow"
app:fiveStarsView_starRating="5"
app:fiveStarsView_changeable="true"
app:fiveStarsView_filledStarDrawable="@drawable/ic_baseline_star_24"
app:fiveStarsView_outlineStarDrawable="@drawable/ic_baseline_star_outline_24"
/>
Anyway, the value which passed by the attributes can be read by TypedArray
like below.
init {
val typedArray = context.obtainStyledAttributes(attrs, R.styleable.FiveStarsView)
typedArray.getDimensionPixelSize(R.styleable.FiveStarsView_fiveStarsView_starMargin, 0)
}
Two-way data binding with custom view
FiveStarsView provides a two-way data binding for convenient use.
To apply it to fiveStarsView_starRating
attribute, the code below is required.
@BindingAdapter("fiveStarsView_starRating")
fun setStarRating(view: FiveStarsView, starRating: Float) {
view.setStarRating(starRating)
}
@InverseBindingAdapter(attribute = "fiveStarsView_starRating")
fun getStarRating(view: FiveStarsView): Float {
return view.getStarRating()
}
The point is the getStarRating()
with @InverseBindingAdapter
method is added for two-way data binding. In this function, it is required to return the value of starRating by FiveStarsView.
🐈⬛ Github Repository
https://github.com/hanbikan/FiveStarsView
GitHub - hanbikan/FiveStarsView: ⭐️ A simple and flexible FiveStarsView, with customizable star images, color, size, and the
⭐️ A simple and flexible FiveStarsView, with customizable star images, color, size, and the like. - GitHub - hanbikan/FiveStarsView: ⭐️ A simple and flexible FiveStarsView, with customizable star i...
github.com
References
https://developer.android.com/training/custom-views/create-view
https://developer.android.com/topic/libraries/data-binding/two-way
'Android' 카테고리의 다른 글
[Android] Cleaning gradle using KTS(Kotlin DSL), Version Catalogs(toml) (0) | 2023.03.08 |
---|---|
Why is @get:Rule used instead of @Rule in Kotlin? (0) | 2023.02.23 |
android/nowinandroid 구조 파헤치기 (0) | 2022.11.30 |
[Android] Touch event to move & pinch zoom (0) | 2022.11.30 |
[Android] Saved image is not appearing in gallery immediately (0) | 2022.11.28 |