목록Kotlin (69)
Hanbit the Developer
Kotlin Documentation 시리즈에 대해 Category: Official libraries - Coroutines 문서 링크: https://kotlinlang.org/docs/coroutines-basics.html Your first coroutine fun main() = runBlocking { // this: CoroutineScope launch { // launch a new coroutine and continue delay(1000L) // non-blocking delay for 1 second (default time unit is ms) println("World!") // print after delay } println("Hello") // main coroutine..
Kotlin Documentation 시리즈에 대해 Category: Standard library 문서 링크: https://kotlinlang.org/docs/opt-in-requirements.html API가 실험적이거나 바뀔 예정이라면 opt-in을 붙여 API 사용자에게 이를 알릴 수 있다. Opt in to using API Propagating opt-in opt-in requirement annotation을 통해 opt-in을 지정할 수 있다. 이때 opt-in이 전파되기 때문에 관련 함수 등에 모두 어노테이션을 달아야 한다. // Library code @RequiresOptIn(message = "This API is experimental. It may be changed in t..
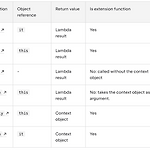
Kotlin Documentation 시리즈에 대해 Category: Standard library 문서 링크: https://kotlinlang.org/docs/scope-functions.html Function selection 각 함수의 의도: Executing a lambda on non-null objects: let val name: String? = "John" name?.let { println("Hello, $it") } // Executes the lambda only if name is non-null Introducing an expression as a variable in local scope: let val result = someObject.let { calculateRes..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/map-operations.html Retrieve keys and values get(), this[key]: 찾을 수 없는 경우 null을 반환한다. getOrElse(), getOrDefault() getValue(): 찾을 수 없는 경우 예외를 던진다. val numbersMap = mapOf("one" to 1, "two" to 2, "three" to 3) println(numbersMap.get("one")) println(numbersMap["one"]) println(numbersMap.getOrDefa..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/set-operations.html union(), intersect(), subtract(): infix fun val numbers = setOf("one", "two", "three") println(numbers union setOf("four", "five")) println(setOf("four", "five") union numbers) println(numbers intersect setOf("two", "one")) println(numbers subtract setOf("three", "four")) ..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/list-operations.html Retrieve elements by index elementAt(), first(), last(), get(), list[index] getOrElse(), getOrNull(): 인덱스를 벗어날 경우 예외를 던지지 않고 따로 처리한다: val numbers = listOf(1, 2, 3, 4) println(numbers.get(0)) println(numbers[0]) //numbers.get(5) // exception! println(numbers.getOrNull(5)) ..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/collection-write.html Adding elements add(), addAll() += val numbers = mutableListOf("one", "two") numbers += "three" println(numbers) numbers += listOf("four", "five") println(numbers) Removing elements remove(), removeAll() retainAll(): removeAll()의 반대. 주어진 컬렉션만을 남긴다. clear() -= val numbers..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/collection-aggregate.html minOrNull(), maxOrNull() average() sum() sumOf() val numbers = listOf(5, 42, 10, 4) println(numbers.sumOf { it * 2 }) println(numbers.sumOf { it.toDouble() / 2 }) 122 30.5 count() 셀렉터를 이용하여 최소값 및 최대값을 검색하는 함수: maxByOrNull(), minByOrNull() maxWithOrNull(), minWithOrNu..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/collection-ordering.html Natural order Comparable을 통해 구현된다. 예시는 다음과 같다: 1은 0보다 크다. b는 a보다 크다. Comparable 인터페이스를 구현하여 compareTo()를 오버라이드 해야 한다. 같은 타입의 객체와 비교하여, 자신의 값이 더 클 경우 양수, 작을 경우 음수, 같을 경우 0을 반환한다. class Version(val major: Int, val minor: Int): Comparable { override fun compareTo(other: ..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/collection-elements.html Retrieve by position *Set은 LinkedHashSet, SortedSet 등 구현 방식에 따라 순서가 바뀐다는 점에 유의하라. elementAt(3) elementAtOrNull(3) elementAtOrElse(5) { index → “The value at $index is undefined” } first() last() Retrieve by condition first {} last {} firstOrNull {}: == find() lastOrNu..