목록Kotlin (69)
Hanbit the Developer
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/collection-parts.html Slice val numbers = listOf("one", "two", "three", "four", "five", "six") println(numbers.slice(1..3)) println(numbers.slice(0..4 step 2)) println(numbers.slice(setOf(3, 5, 0))) [two, three, four] [one, three, five] [four, six, one] Take and drop val numbers = listOf("one..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/collection-grouping.html groupBy()는 람다 함수를 통해 key를 만들어 Map을 반환한다. val numbers = listOf("one", "two", "three", "four", "five") println(numbers.groupBy { it.first().uppercase() }) println(numbers.groupBy(keySelector = { it.first() }, valueTransform = { it.uppercase() })) {O=[one], T=[two, three..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/collection-filtering.html Filter by predicate filter { it → true } filterNot { it → true }: false인 아이템을 반환 filterIndexed { index, item → true } filterIsInstance(): String인 아이템을 반환 filterNotNull(): NotNull인 아이템을 반환 Partition val numbers = listOf("one", "two", "three", "four") val (match, rest)..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/collection-transformations.html Map map, mapIndexed mapNotNull, mapIndexedNotNull: map + null filter mapKeys, mapValues: key 또는 value에 대해서 map을 적용한 Map을 생성한다. val numbersMap = mapOf("key1" to 1, "key2" to 2, "key3" to 3, "key11" to 11) println(numbersMap.mapKeys { it.key.uppercase() }) printl..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/sequences.html Iterable과 유사하지만 lazily하게 작동한다. Sequence는 처리를 하나씩 하지만, Iterable은 모든 컬렉션을 모두 처리한 뒤 다음 절차를 밟는다. 따라서 Sequence를 사용하면, ‘중간 결과물’ 만드는 과정을 제거하여 성능을 향상시킬 수 있다. 하지만 작은 컬렉션이나 간단한 계산에 대해서는 오히려 오버헤드를 증가시킬 수 있다. 따라서 상황에 맞게 Sequence 또는 Iterable을 사용해야 한다. ⇒ 컬렉션이 작거나 계산이 단순할 경우 Iterable, 아닌 경우 S..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/ranges.html for (i in 1..4) print(i) for (i in 4 downTo 1) print(i) for (i in 1..8 step 2) print(i) println() for (i in 8 downTo 1 step 2) print(i) for (i in 1 until 10) { // i in 1 until 10, excluding 10 print(i) } ..는 operator fun rangeTo()과 같다. step, downTo는 infix fun이며, IntProgression 등을 ..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/iterators.html 구체적인 자료 구조를 알지 못해도 연속된 아이템을 돌 수 있는 메커니즘을 제공한다. 아이템들을 출력하거나, 모든 아이템의 부호를 바꾸는 등의 작업을, 컬렉션의 종류와 상관 없이, 하나의 함수로 구현이 가능하다. Iterable을 통해 제공되는 iterator()를 통해 반복자에 접근할 수 있다. val numbers = listOf("one", "two", "three", "four") val numbersIterator = numbers.iterator() while (numbersItera..
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/constructing-collections.html Create with collection builder functions val map = buildMap { // this is MutableMap, types of key and value are inferred from the `put()` calls below put("a", 1) put("b", 0) put("c", 4) } println(map) // {a=1, b=0, c=4} Initializer functions for lists val doubled..
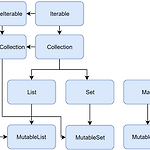
Kotlin Documentation 시리즈에 대해 Category: Standard library - Collections 문서 링크: https://kotlinlang.org/docs/collections-overview.html Collection types 각 인터페이스는 아래의 타입으로 나뉜다. read-only collection covariant(out) mutable collection 굳이 var를 쓰지 않아도 된다.(대입 연산자가 아니라 메소드를 통해 컬렉션을 수정하므로) covariant가 아니다. Collection 읽기 전용 컬렉션의 공통 행위를 정의하며, List와 Set의 상위 인터페이스이다. fun printAll(strings: Collection) { for(s in st..
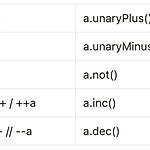
Kotlin Documentation 시리즈에 대해 Category: Concepts - Functions 문서 링크: https://kotlinlang.org/docs/operator-overloading.html interface IndexedContainer { operator fun get(index: Int) } class OrdersList: IndexedContainer { // operator modifier is omitted because get() overrides operator fun override fun get(index: Int) { /*...*/ } } Unary operations data class Point(val x: Int, val y: Int) operator f..